Alright, so today I’m gonna walk you through this thing I messed around with called “dart shirt.” Sounds kinda weird, I know, but trust me, it’s kinda cool. Basically, I wanted to see if I could whip up a simple command-line tool using Dart to, like, generate some basic boilerplate code for different shirt sizes. Yeah, I know, super niche, but hey, gotta start somewhere, right?
So, first things first, I fired up my trusty VS Code and made a new Dart project. Nothing fancy, just a plain ol’ console application. I named it “dart_shirt,” because, well, why not?
Next up, I had to figure out what I actually wanted the thing to do. I decided I wanted it to take a shirt size (like S, M, L, XL) as input and then spit out some basic dimensions, like chest width, shoulder width, and maybe sleeve length. Just ballpark figures, nothing super precise. This wasn’t gonna be a bespoke tailoring app, just a quick-and-dirty code generator.
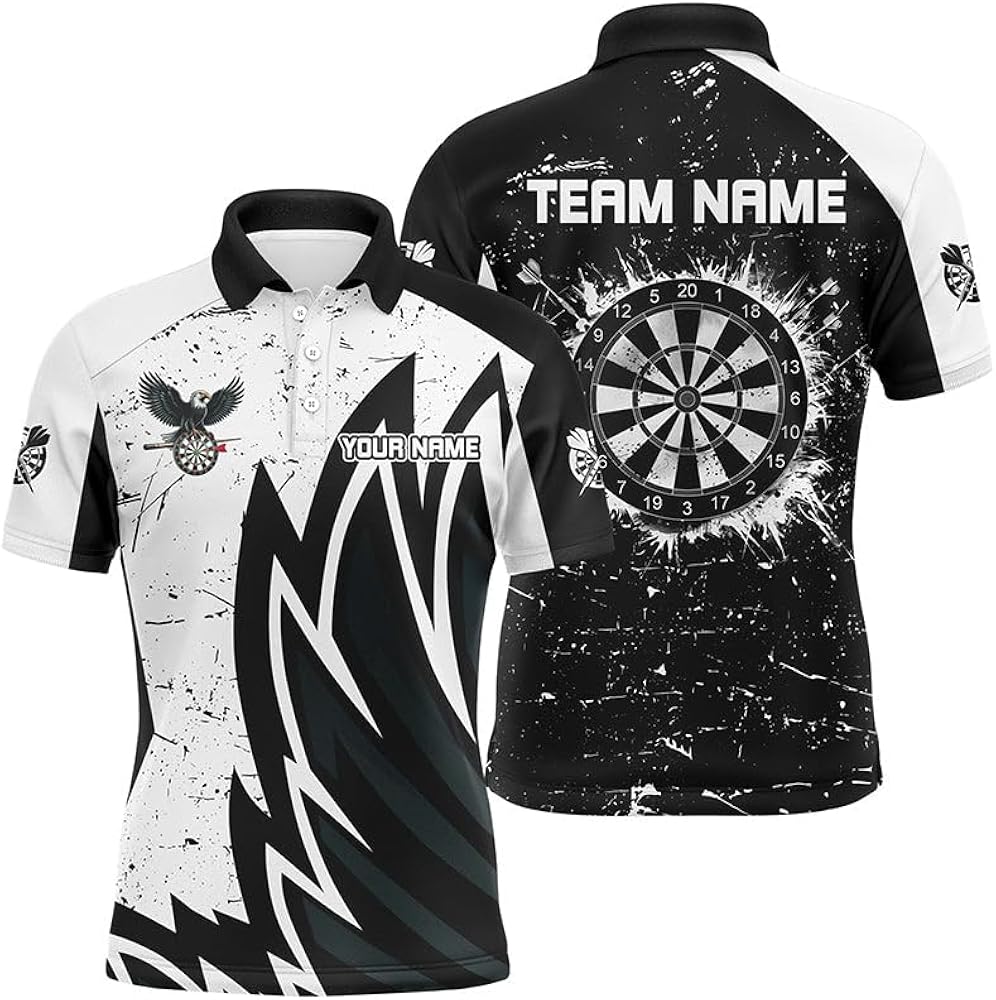
I started by defining a simple `enum` for the shirt sizes:
dart
enum ShirtSize {
S,
M,
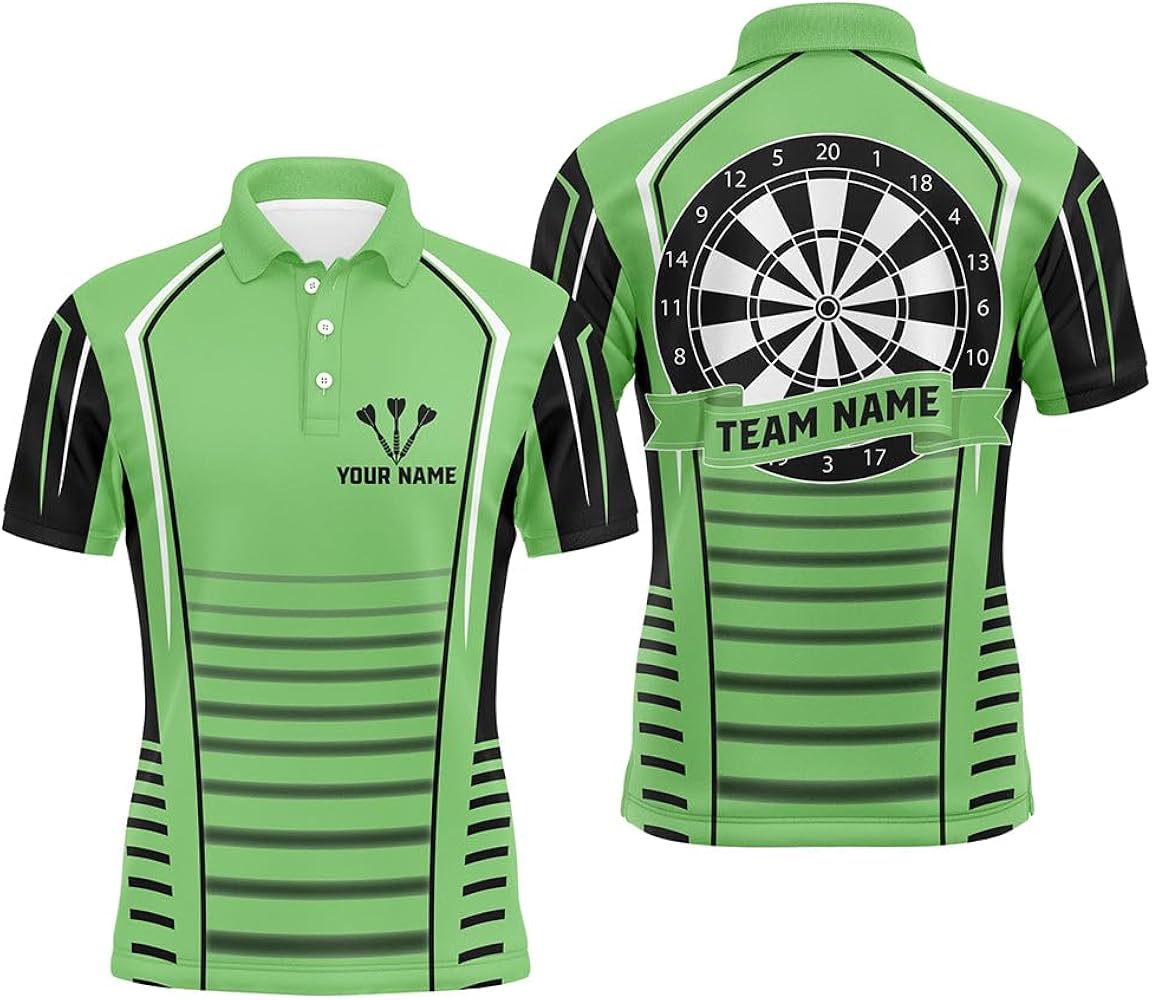
L,
XL,
XXL
Pretty straightforward, right? Then I needed a way to map those sizes to actual dimensions. I figured a simple `Map` would do the trick:
dart
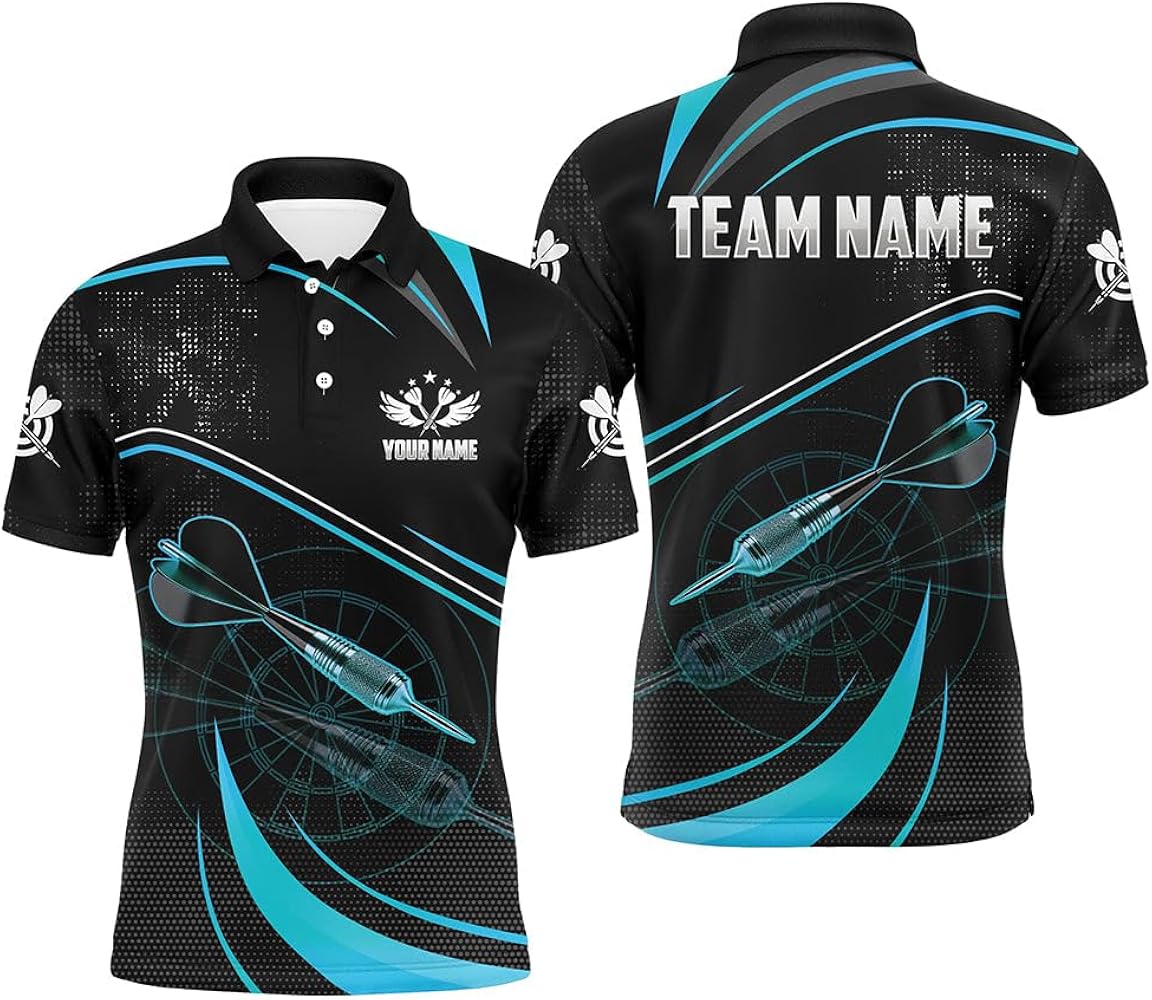
final shirtDimensions = {
ShirtSize.S: {‘chest’: 36, ‘shoulder’: 16, ‘sleeve’: 24},
ShirtSize.M: {‘chest’: 40, ‘shoulder’: 18, ‘sleeve’: 25},
ShirtSize.L: {‘chest’: 44, ‘shoulder’: 20, ‘sleeve’: 26},
*: {‘chest’: 48, ‘shoulder’: 22, ‘sleeve’: 27},
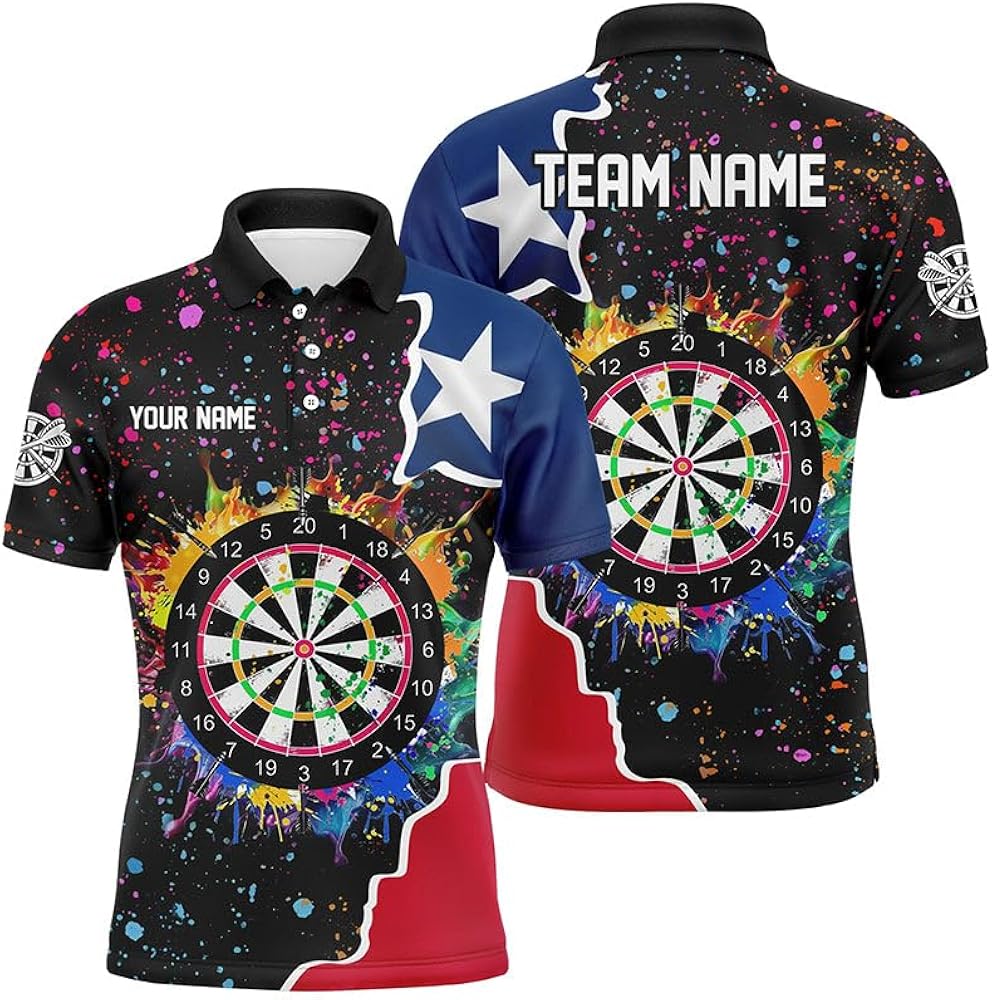
*: {‘chest’: 52, ‘shoulder’: 24, ‘sleeve’: 28},
Again, super basic. Just some made-up numbers for demonstration purposes.
Now for the meat of the thing: the command-line interface. I used the `args` package to handle parsing the input. Added it to my `*`:
yaml
dependencies:
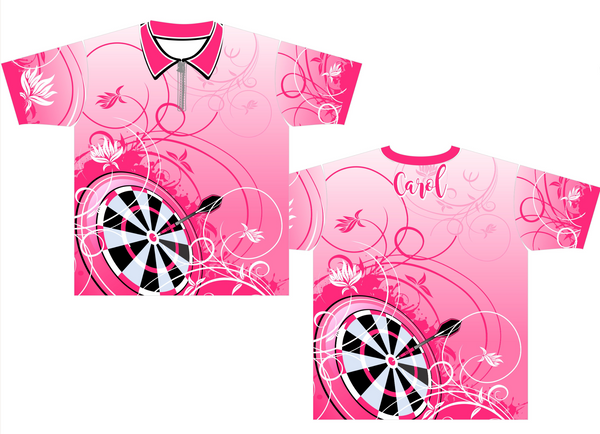
args: ^2.4.0
Then I ran `dart pub get` to pull it in. With that in place, I could set up the argument parser:
dart
import ‘package:args/*’;
void main(List arguments) {
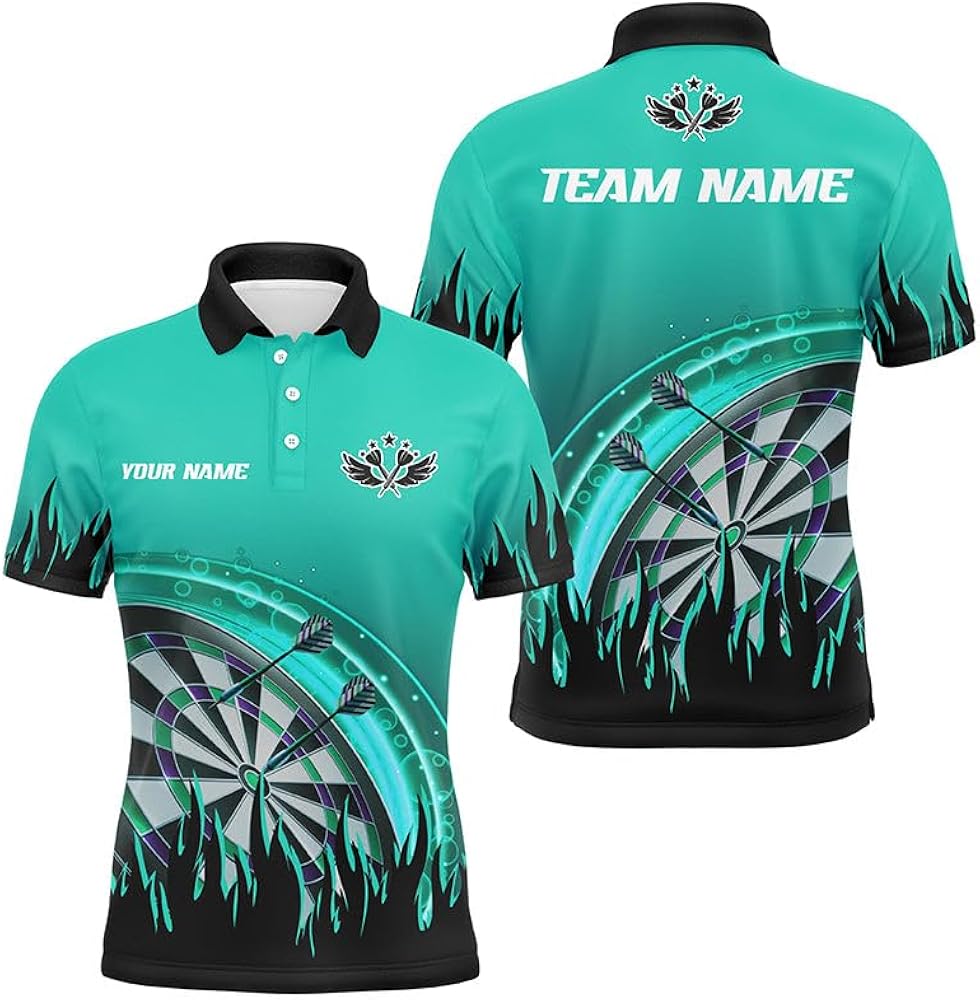
final parser = ArgParser();
*(‘size’, abbr: ‘s’, mandatory: true, help: ‘Shirt size (S, M, L, XL, XXL)’);
try {
final results = *(arguments);
final sizeString = results[‘size’];
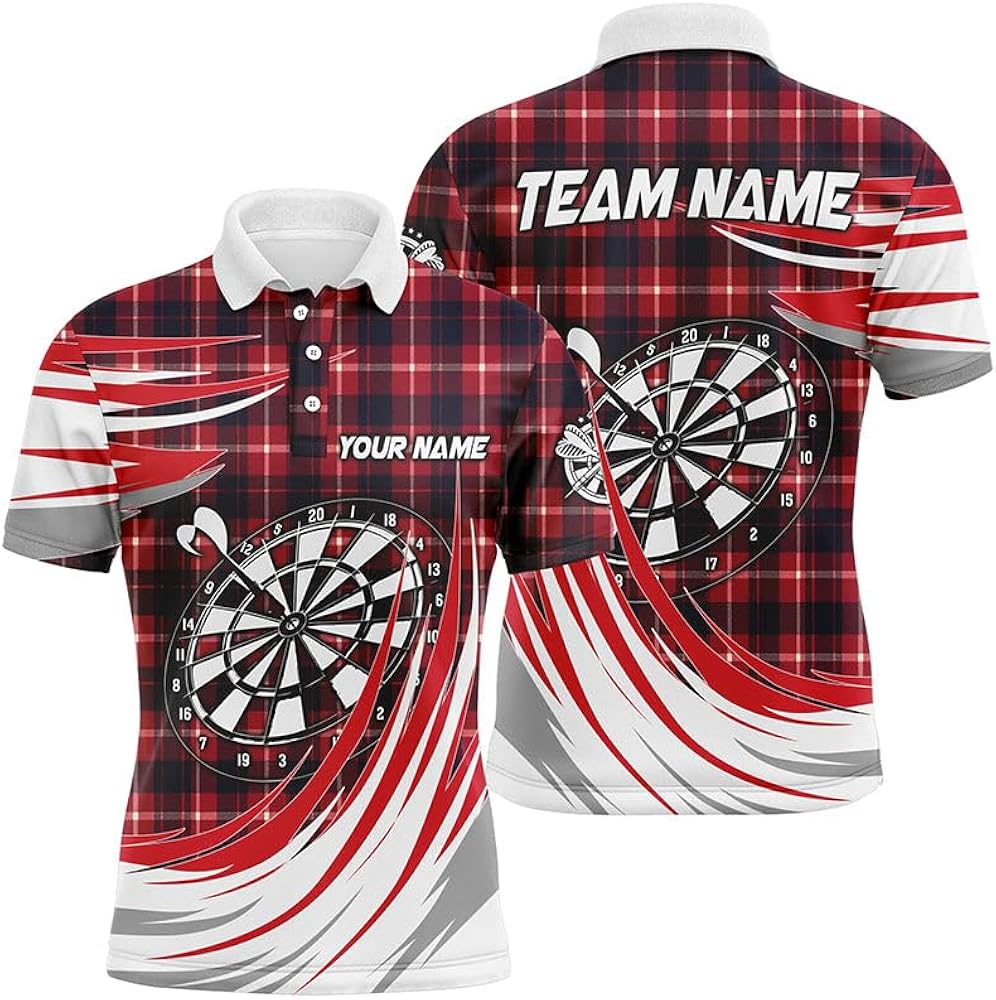
ShirtSize size;
switch (*()) {
case ‘S’:
size = ShirtSize.S;
break;
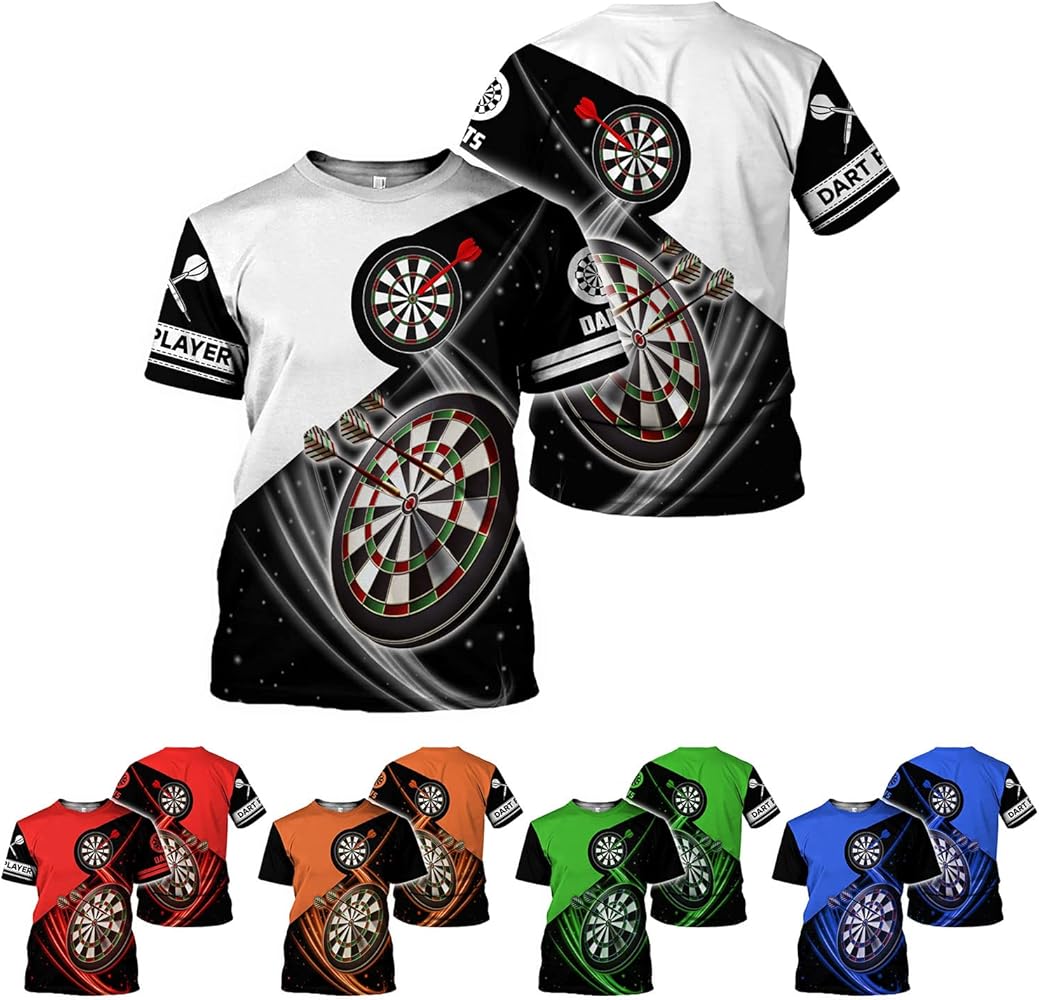
case ‘M’:
size = ShirtSize.M;
break;
case ‘L’:
size = ShirtSize.L;
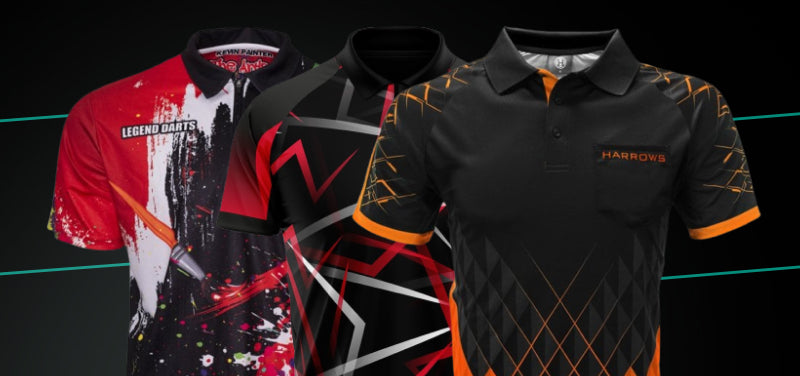
break;
case ‘XL’:
size = *;
break;
case ‘XXL’:
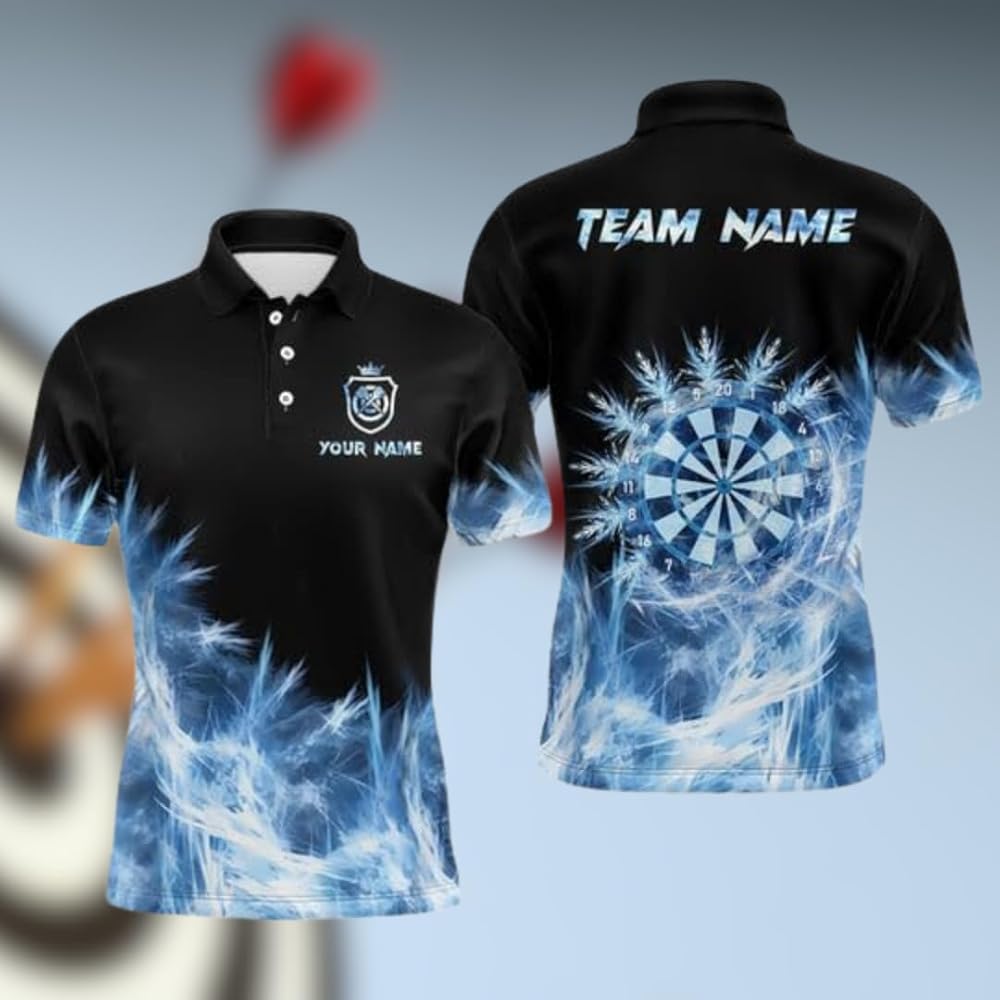
size = *;
break;
default:
throw FormatException(‘Invalid shirt size: $sizeString’);
final dimensions = shirtDimensions[size];
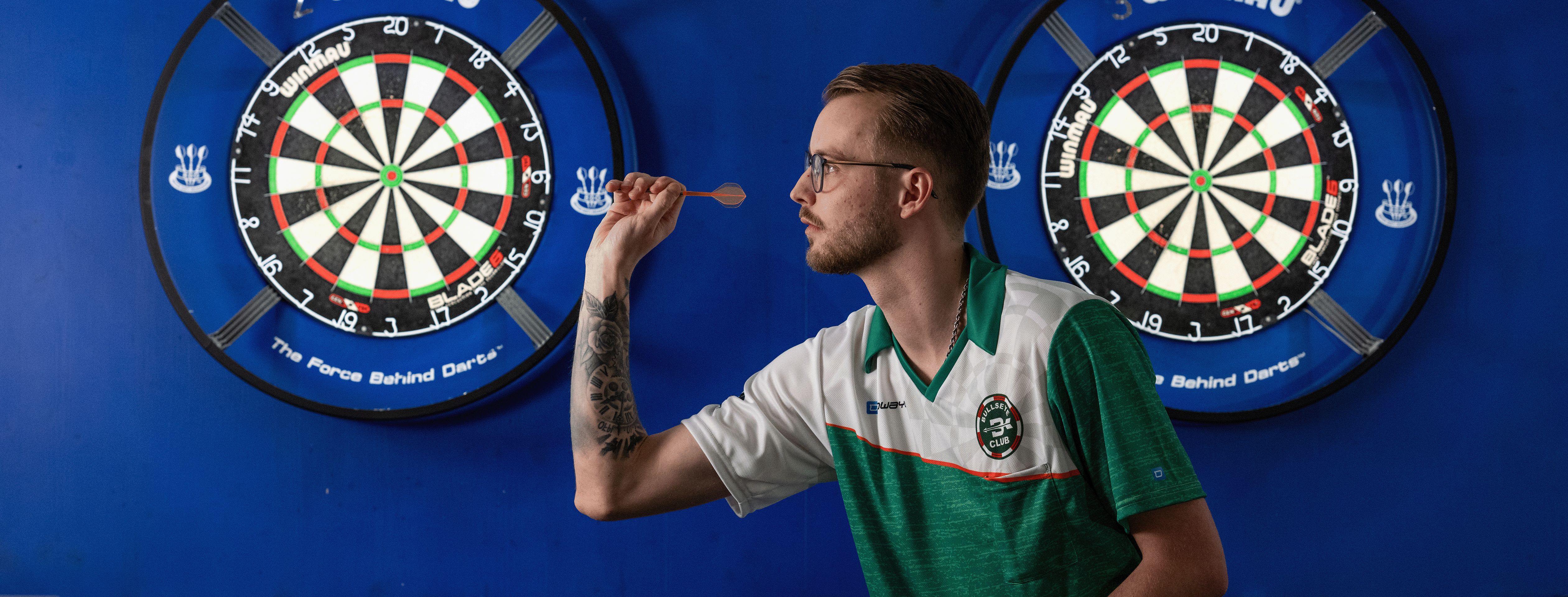
if (dimensions != null) {
print(‘Shirt size: $size’);
print(‘Chest width: ${dimensions[‘chest’]} inches’);
print(‘Shoulder width: ${dimensions[‘shoulder’]} inches’);
print(‘Sleeve length: ${dimensions[‘sleeve’]} inches’);
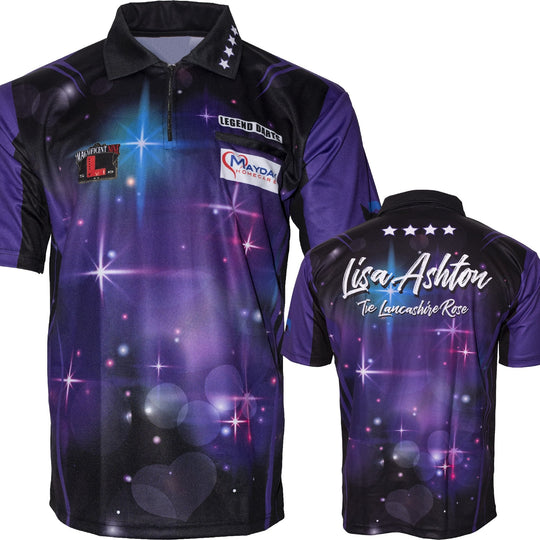
} else {
print(‘Dimensions not found for size: $size’);
} catch (e) {
print(e);
print(*);
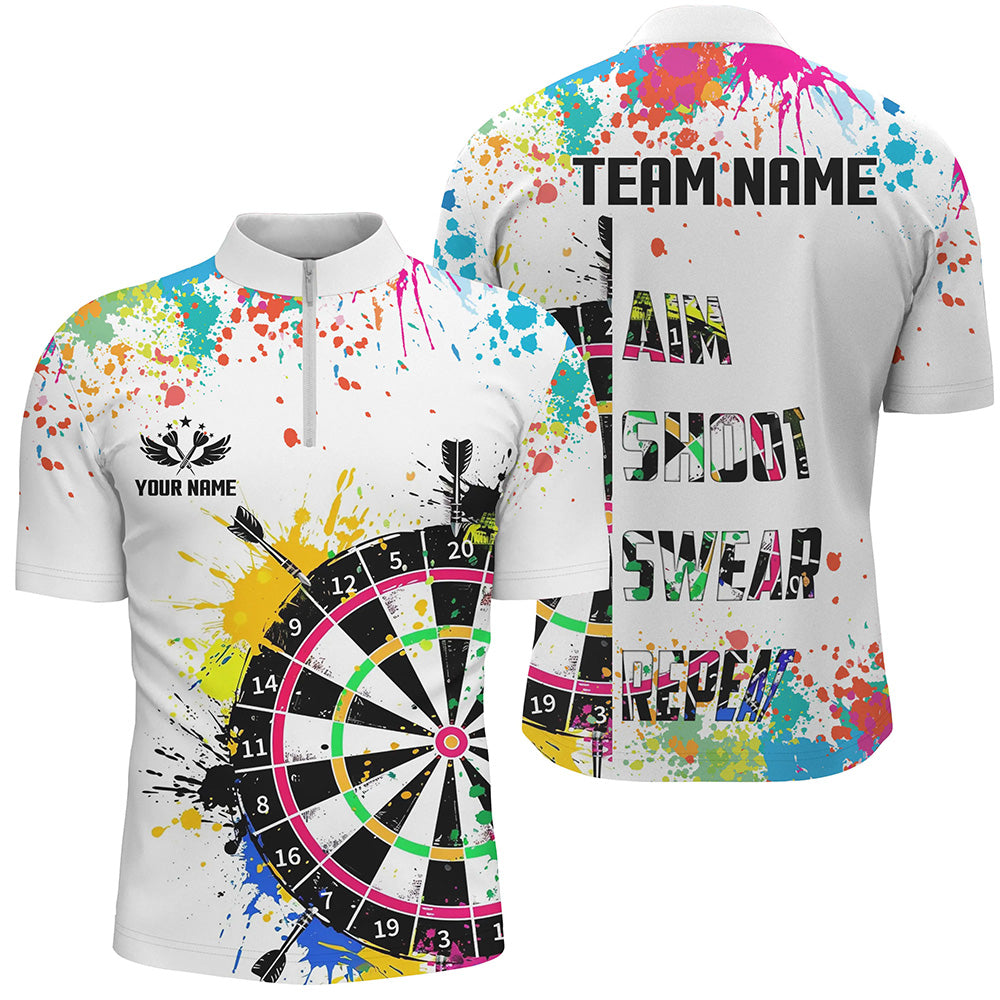
Okay, let’s break that down. I created an `ArgParser` and added an option called “size” with a short alias of “s.” I made it mandatory because, duh, you need a size to generate dimensions. Then, in the `try` block, I parsed the arguments and converted the size string to a `ShirtSize` enum value. After that, I looked up the dimensions in my `shirtDimensions` map and printed them out. If anything went wrong (like an invalid size), I caught the exception and printed an error message along with the parser’s usage instructions. Handy, right?
Finally, I ran it from the command line. Something like:
bash
dart run dart_* -s L
And it spat out:
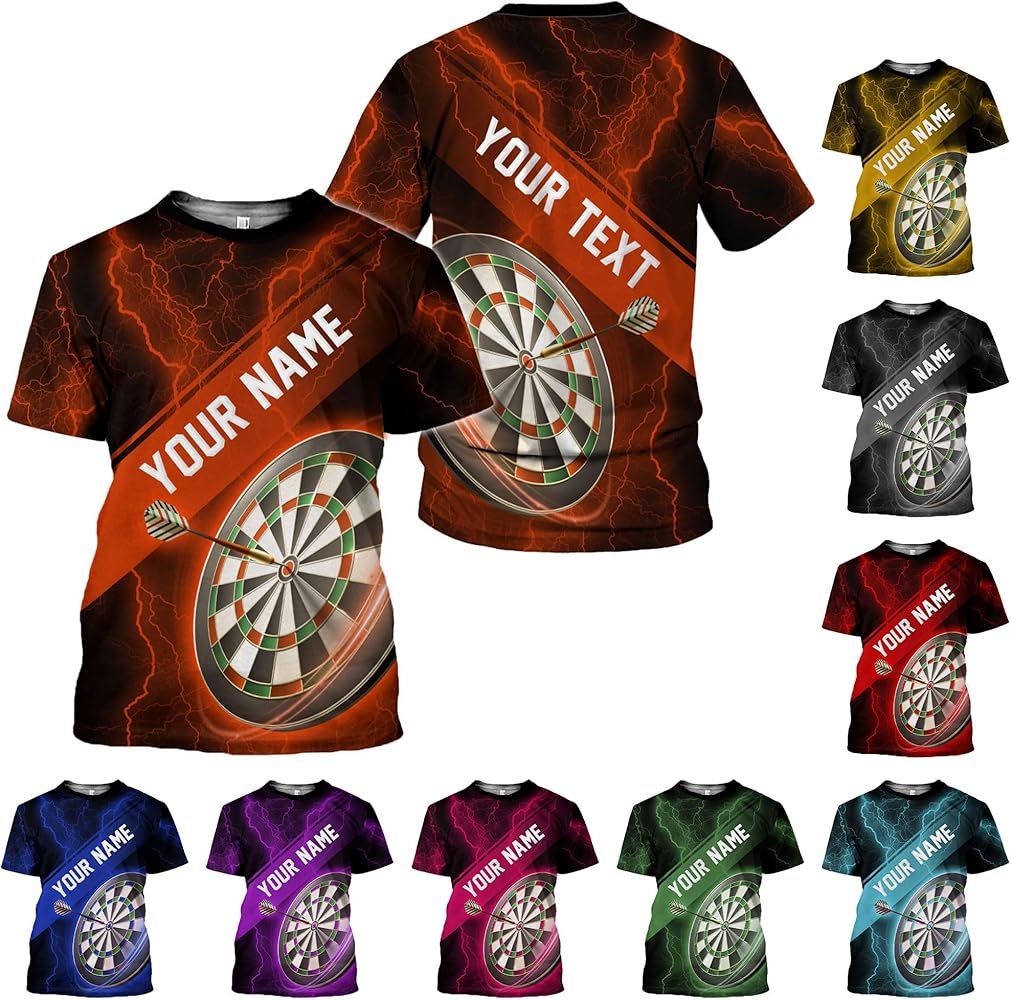
Shirt size: ShirtSize.L
Chest width: 44 inches
Shoulder width: 20 inches
Sleeve length: 26 inches
Boom! It worked! Okay, it wasn’t exactly rocket science, but it was a fun little project. I learned a bit about using enums, maps, and the `args` package in Dart. Plus, now I have a super-niche tool that… well, I’m not sure what I’ll use it for, but it’s there if I need it! Maybe I’ll expand it later to generate different types of clothing or something. Who knows?
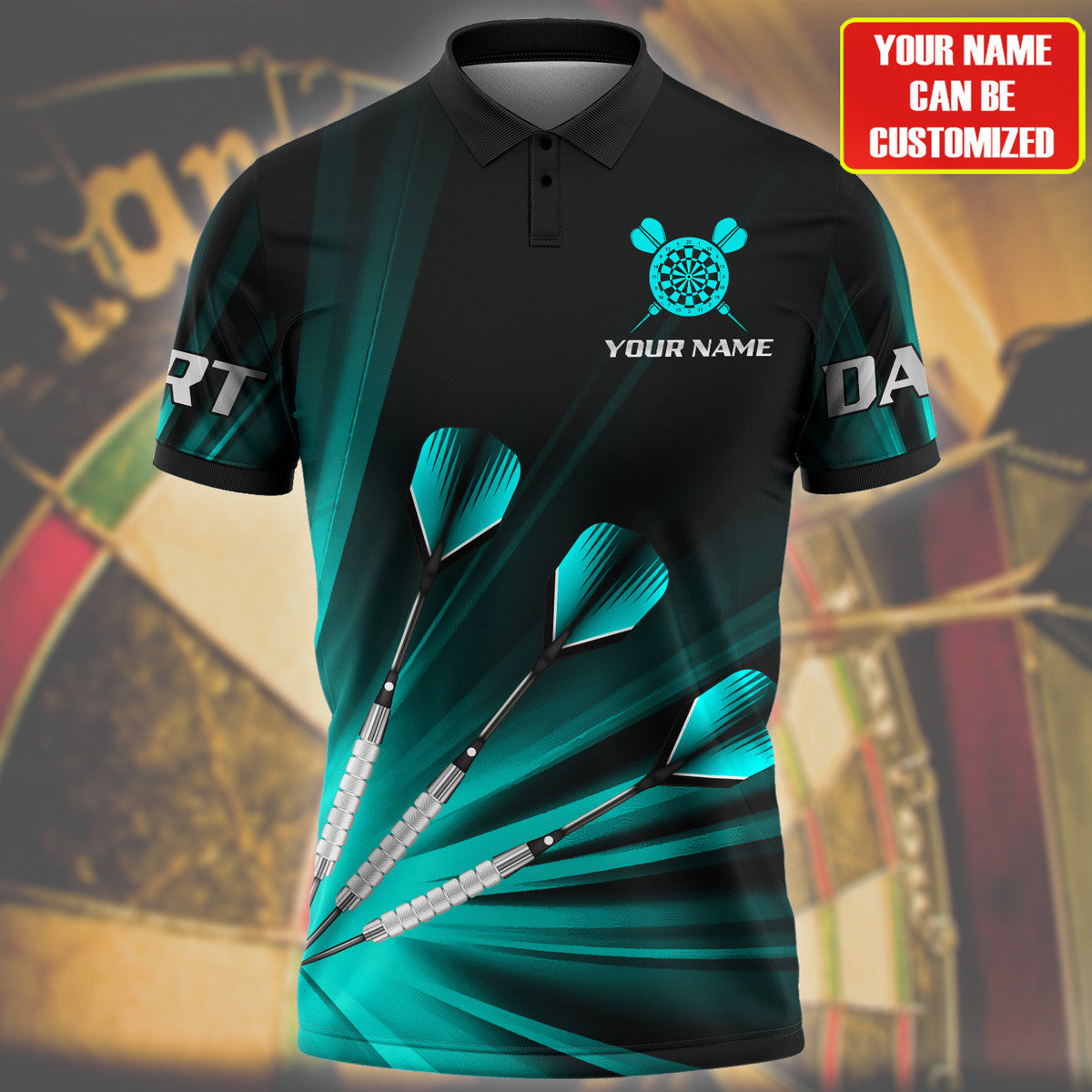
Anyway, that’s my “dart shirt” adventure. Hope you found it somewhat interesting. Maybe it’ll inspire you to try something similar. Just pick a random idea and run with it. You might be surprised what you come up with.