Alright, so I decided to tackle this “kd bucket list” thing. I’d heard about it and it sounded interesting, a way to organize my learning, so I dove in.
First, I had to figure out what the heck it even was. The title “kd bucket list” that I had in mind, but after searching the net, it became apparent it was all about using Kubernetes (k8s) and Docker. Okay, cool, I’ve dabbled with Docker before, and Kubernetes is something I’ve been meaning to learn.
I started by just creating a simple text file. I just named it `*`. My initial plan was pretty basic: just list some things I wanted to learn or do related to Kubernetes.
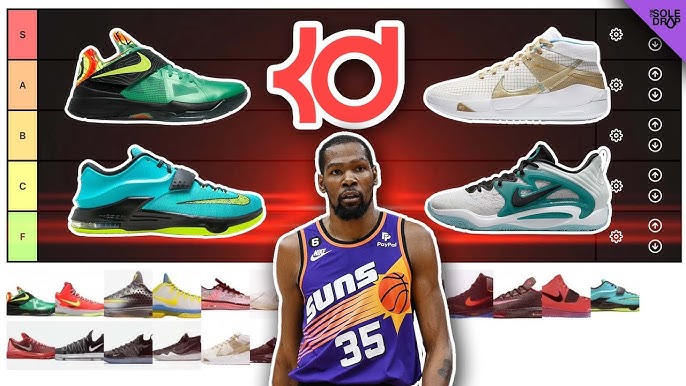
My list looked something like this:
- Set up a basic Kubernetes cluster.
- Deploy a simple “Hello, World!” app.
- Figure out how to scale that app.
- Learn about services and networking in Kubernetes.
Then, it dawned that i need to create Dockerfile. I remembered the basic structure – you know, `FROM`, `WORKDIR`, `COPY`, `RUN`, `CMD` – those kinda things. My Dockerfile was super simple, something like:
dockerfile
FROM python:3.9-slim
WORKDIR /app
COPY * .
RUN pip install –no-cache-dir -r *
COPY . .
CMD [“python”, “*”]
I used Python because, I’m kinda comfortable with it.
Of course, I got a `*`. It’s also super simple. Flask is included.
Flask==2.0.0
Then, built the Docker image. I ran `docker build -t my-bucket-list-app .` in the terminal. And waited.
Next, I wanted to test it before going into the Kubernetes craziness. So I ran `docker run -p 5000:5000 my-bucket-list-app`. Opened my browser, typed in `localhost:5000`, and… boom! My super simple app was there, looking beautiful.
Kubernetes Time!
I used minikube to create a local Kubernetes cluster. I installed it before using the brew command. It seemed like the easiest way to get started without setting up a whole cloud environment. Ran `minikube start` and crossed my fingers.
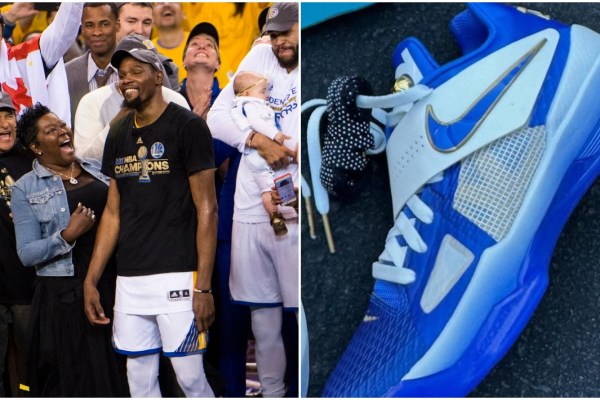
After a bit of waiting, minikube was up and running. Now for the scary part: deploying to Kubernetes.
I created a Kubernetes deployment YAML file (`*`). This was a bit of a learning curve. I basically copy-pasted from the Kubernetes documentation and tweaked it for my app. Things like `apiVersion`, `kind`, `metadata`, `spec`, `replicas`, `selector`, `template`… yeah, lots of new stuff.
yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: bucket-list-deployment
spec:
replicas: 3
selector:
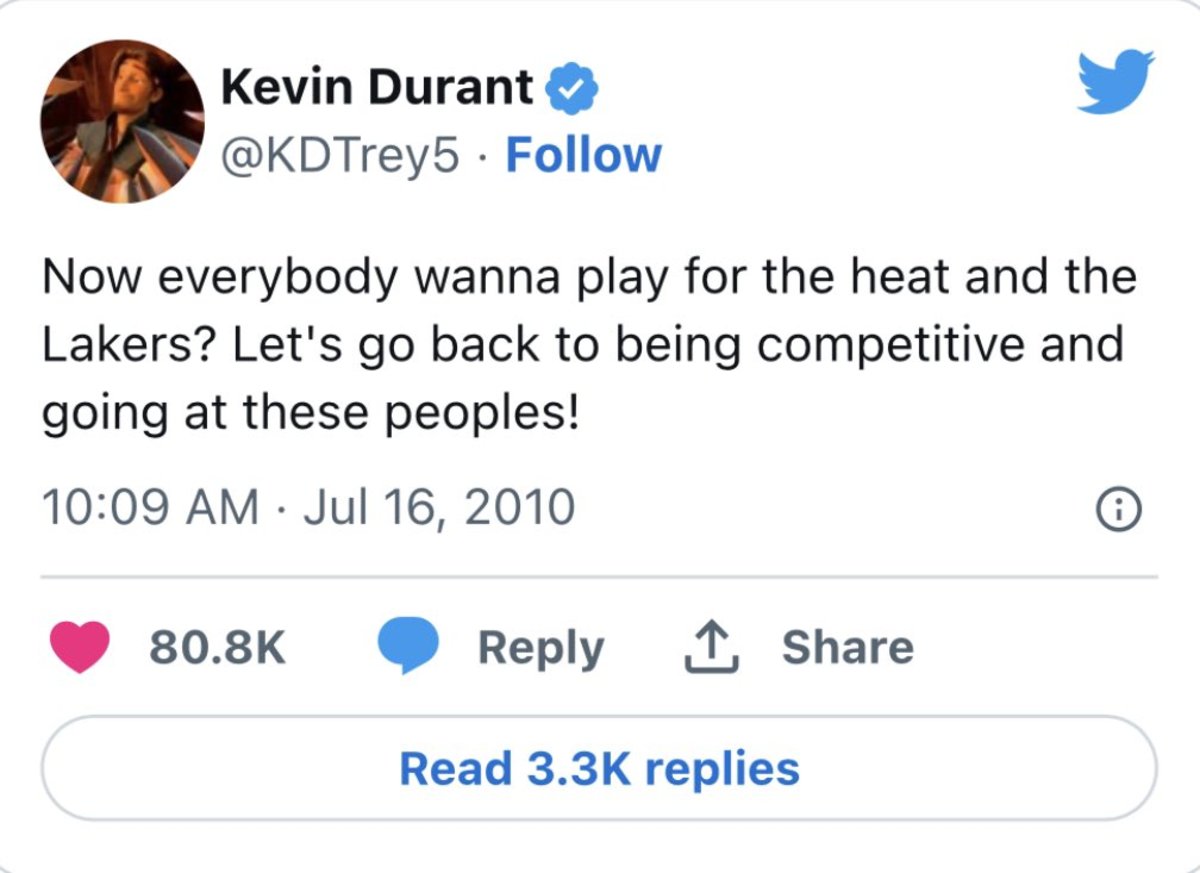
matchLabels:
app: bucket-list
template:
metadata:
labels:
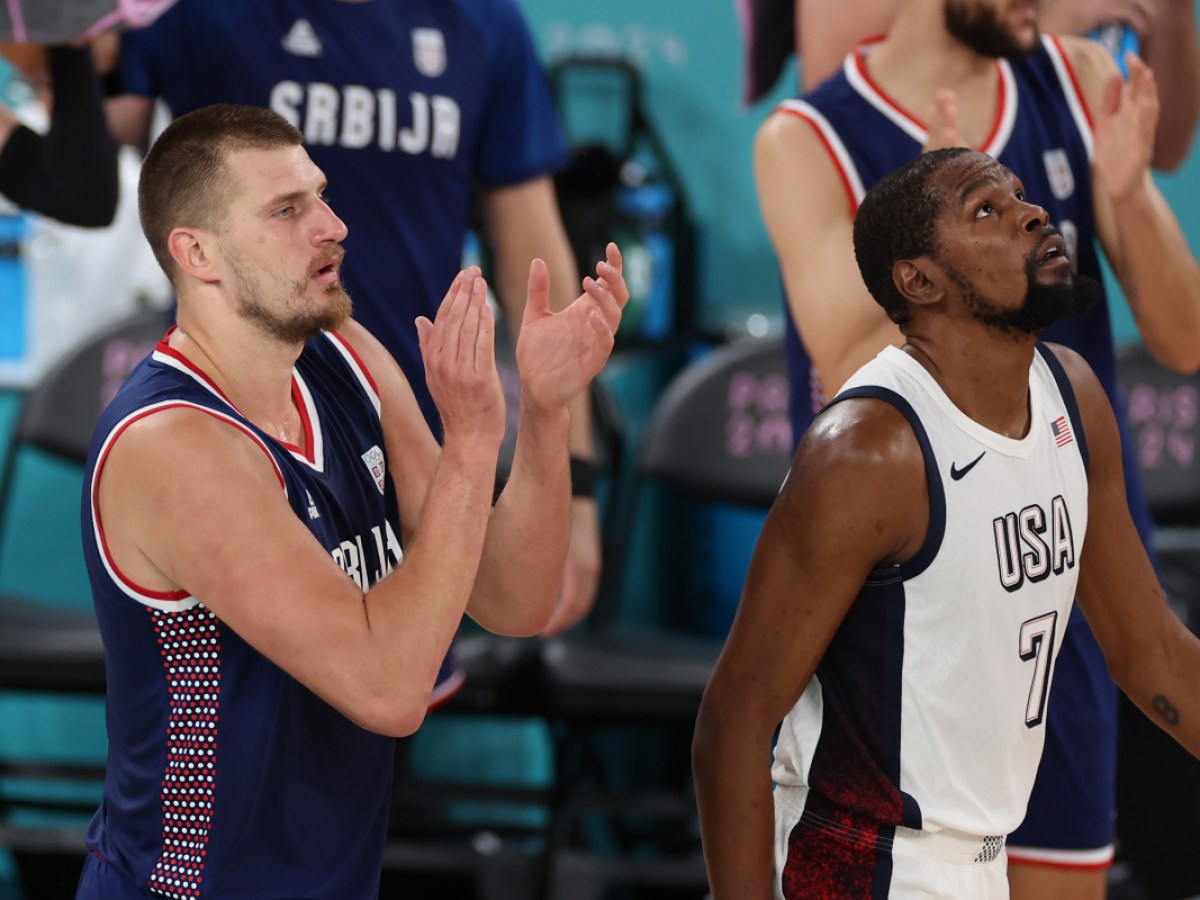
app: bucket-list
spec:
containers:
– name: bucket-list-container
image: my-bucket-list-app:latest # Use your image name here
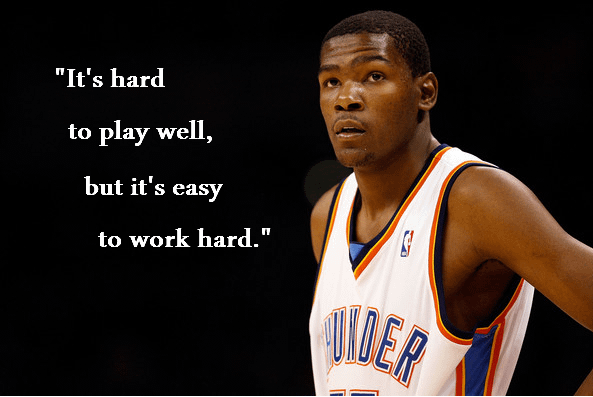
ports:
– containerPort: 5000
Then I created a service YAML file (`*`) to expose the deployment. More YAML fun! This one defined how to access my app from outside the cluster.
yaml
apiVersion: v1
kind: Service
metadata:
name: bucket-list-service
spec:
selector:
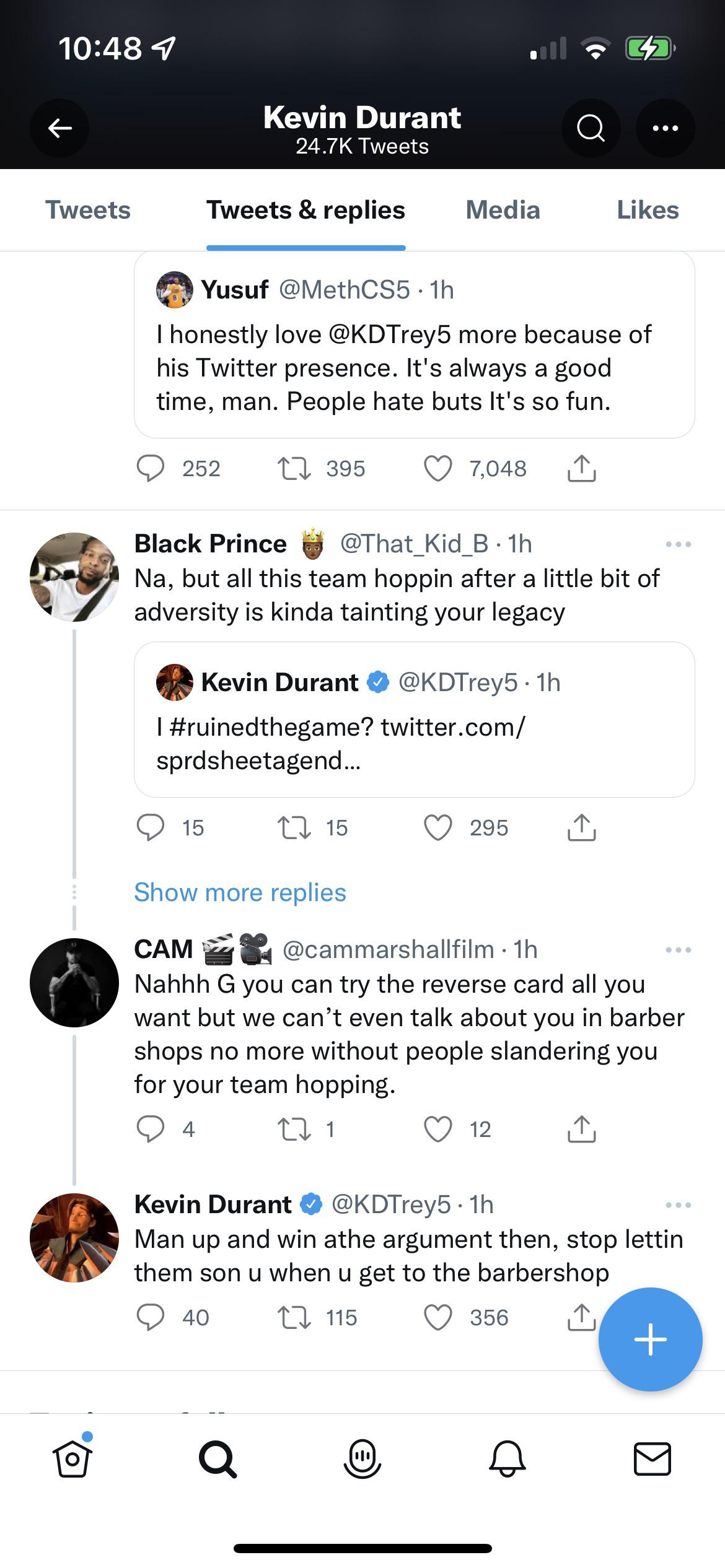
app: bucket-list
ports:
– protocol: TCP
port: 80
targetPort: 5000
type: LoadBalancer
I applied these YAML files using `kubectl apply -f *` and `kubectl apply -f *`. Then I checked if everything was running with `kubectl get pods` and `kubectl get services`.
Finally, I got the URL for my app using `minikube service bucket-list-service –url`. Opened that URL in my browser, and… there it was! My little app, running in Kubernetes!
It was a lot of steps, and a lot of new concepts, but it felt good to actually get something working. It’s like going from zero to… well, maybe 0.1 in the world of Kubernetes, but it’s a start! Next is the auto-scaling and persistent storage, I think.