Okay, so I wanted to build a simple contact management system. I’ve heard Go is pretty efficient for this kind of thing, so I thought, “Why not give it a shot?” I’m calling it “gojo contacts” – kinda catchy, right?
First, I set up my Go workspace. You know, the usual: making sure my GOPATH
is set correctly and all that jazz. I created a new directory for my project, ‘gojo-contacts’.
Planning the Structure
Next, I thought about the structure. What do I actually need? Well, obviously, a way to store contact information. I decided on a simple struct for each contact:
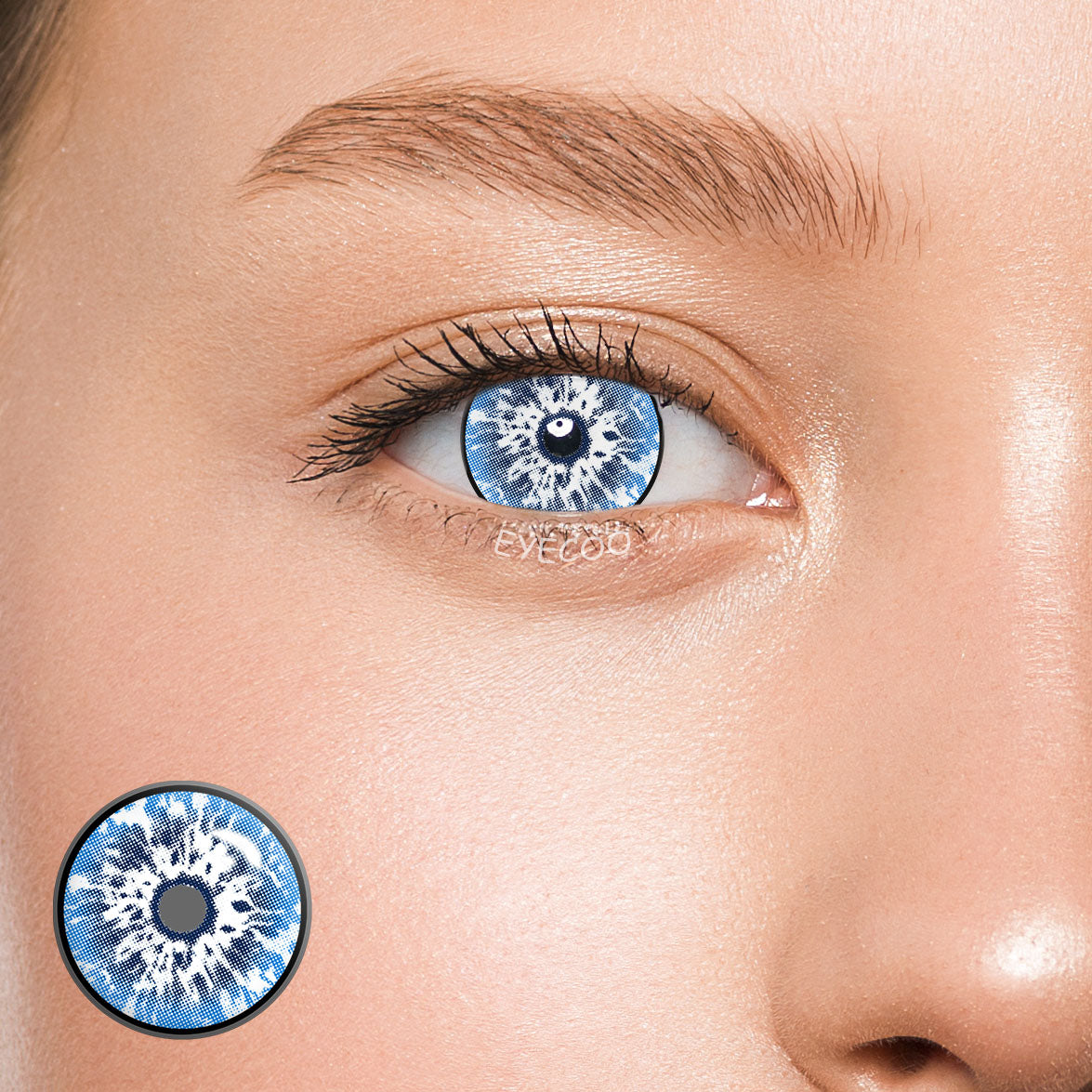
- Name (string)
- Email (string)
- Phone Number (string)
Initially I used slice to store contacts.
Coding the Basics
Then came the coding part. I started with the basic operations:
- Adding a contact: Created a function to take input for the name, email, and phone number, then create a new contact struct, and store the data.
- Displaying all contacts: Wrote a function to simply loop through my contacts and print their details. Very basic formatting, just to see if it’s working.
Iterate, iterate…
After finishing the basic function, I try to make it more efficient.
- Change the storage from slice to map: I think use map will make the search faster.
- Add Delete function: Input the contact name, delete it from the map.
- Add Search function: Input part of the name, display the match result.
The End (For Now!)
So, that’s where I’m at. “gojo contacts” is super basic right now, but it does what it’s supposed to do. I can add contacts, see them all, and search. It was a fun little project to get my hands dirty with Go, and it definitely gave me a good starting point for building something more complex in the future. It’s a never-ending journey of learning, and I like it!