Okay, so today I’m diving into this “tournament rankings nyt” thing. I saw it floating around and figured, why not give it a shot? Sounds kinda fun, right?
First off, I started by trying to really understand the problem. Like, what exactly are we trying to rank here? Is it a single-elimination tournament? Round-robin? A mix? The details are key, ya know?
Next, I grabbed my weapon of choice: Python. It’s just my go-to for messing around with stuff like this. I like to keep it simple, at least at the start. So, I created a basic Python file and started outlining what I needed.
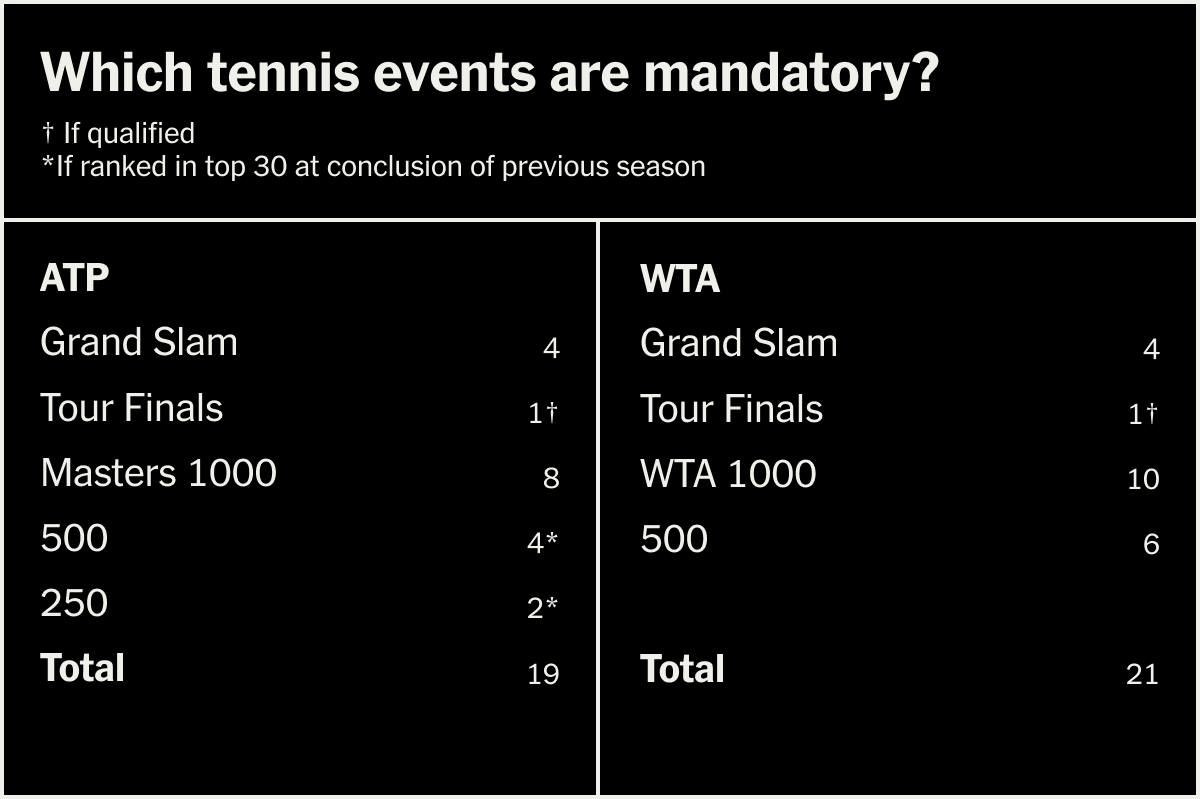
Here’s the rough plan:
- Read in the tournament data (names, wins, losses, scores whatever is available).
- Figure out some ranking criteria. Maybe wins are the primary thing, then point difference or something.
- Implement the ranking logic. Get a list of players sorted based on the ranking criteria.
- Output the rankings. Simple as that.
Okay, so the reading data part, that’s where I actually got stuck for a bit. I needed some data, right? But I didn’t have a pre-made dataset or anything. So, I started with a super simple example. Like, just four players, three rounds. Nothing fancy at all.
I literally just typed it out as a list of dictionaries in my Python file. Crude, yes, but it worked for testing. After that, I did some test data input and output.
Then comes the ranking logic. I spent a bit of time playing around with different ranking algorithms. I looked at a couple of different options, like:
- Simple wins: The player with the most wins is ranked highest.
- Win percentage: Wins divided by total matches played.
- Elo rating: Elo is a ranking system that considers the relative skill levels of the players.
I initially went with a simple “wins first, then point difference” approach. That felt reasonably intuitive. I wrote a function to calculate the point difference (points scored – points against), and another function to actually do the sorting. Python’s `sorted()` function with a `lambda` key is your friend here!
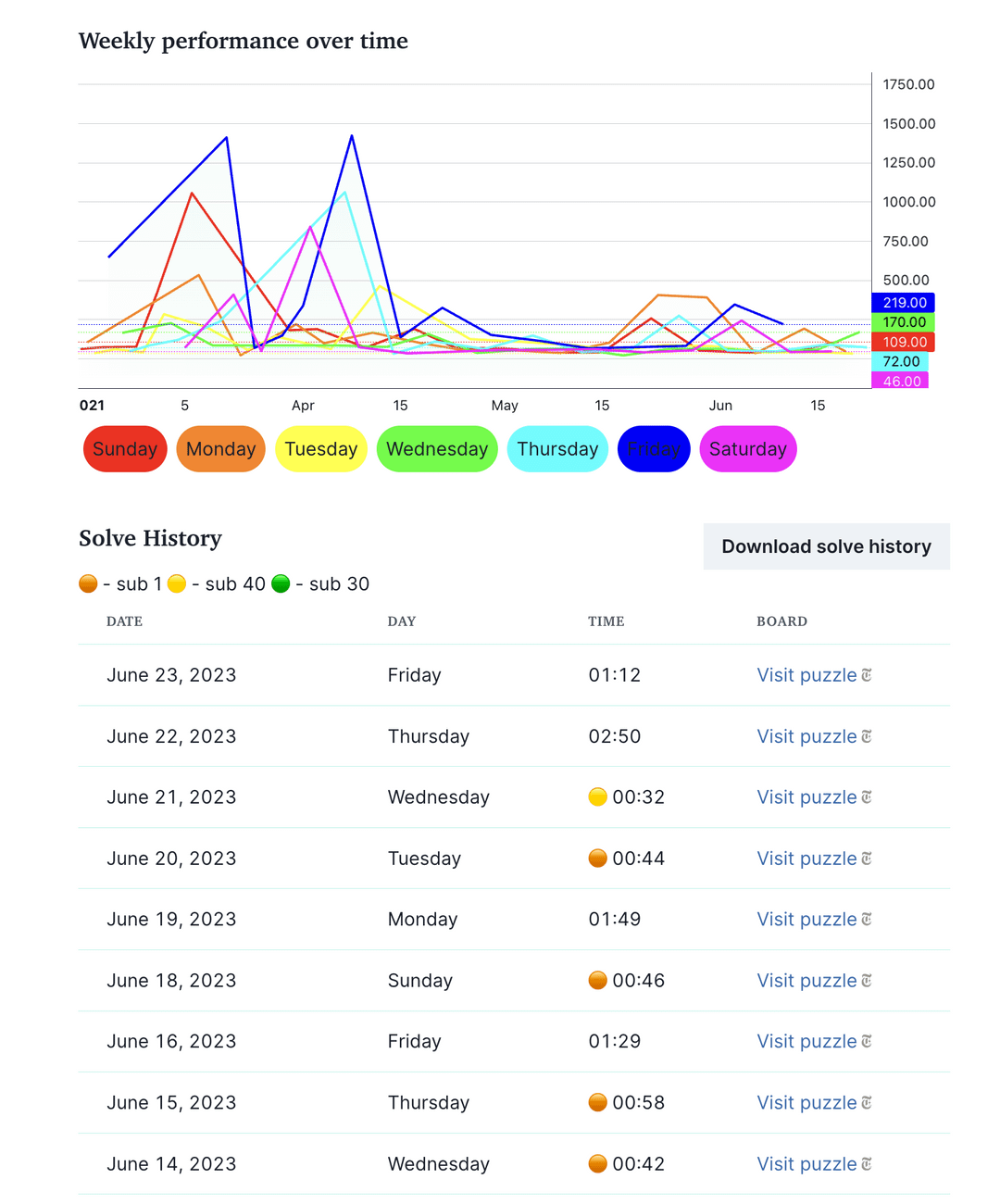
The hardest part of the entire process was figuring out how to visualize the output. I initially just printed it out to the terminal, which wasn’t the prettiest thing in the world. Eventually, I had to create a table output, which was more readable.
After tweaking a bit and adding some comments to the code, the tournament rankings were working well. It might not be the most perfect code, but it does what it needs to do and is easy to understand.