Okay, so today I’m gonna share my experience building this little thing I call “dart town.” It’s nothing crazy, just a fun project I did to kinda get my head around Dart and Flutter a little better.
It all started when I was messing around with Flutter and kept seeing these cool UI demos. I was like, “I wanna make something that actually does something, not just look pretty.” So, I decided on a simple idea: a place where you can add “buildings” (just text fields, really), and they show up on a screen, like a little town.
First things first, I fired up a new Flutter project. I used the command line, you know, flutter create dart_town
. Standard stuff. Then came the fun part – figuring out the basic layout. I knew I wanted a way to add new buildings, and a main area to display them.

I started with a simple Scaffold
. Inside, I added an AppBar
for the title. Below that, I put a Column
. This Column
would hold two main things: the input area for adding buildings, and the display area.
For the input area, I used a Row
with a TextField
for the building name and a ElevatedButton
that says “Add Building.” Hooking up the onPressed
function of the button was a bit tricky at first. I had to create a stateful widget so I could manage the list of buildings and update the UI when a new one was added.
Speaking of the list of buildings, I used a List to store the names. Every time the "Add Building" button is pressed, the text from the
TextField
gets added to this list. Then, I call setState
to rebuild the UI.
The display area was just a . It takes the list of building names and displays each one as a simple Card
with the name inside. I added a little padding and margin to make them look nicer.
Now, here's where things got a little interesting. I wanted to be able to remove buildings. So, I wrapped each Card
in a Dismissible
widget. This lets you swipe a building to the side to delete it. The onDismissed
callback is where the magic happens. I remove the building from the list, and again, call setState
to update the UI.
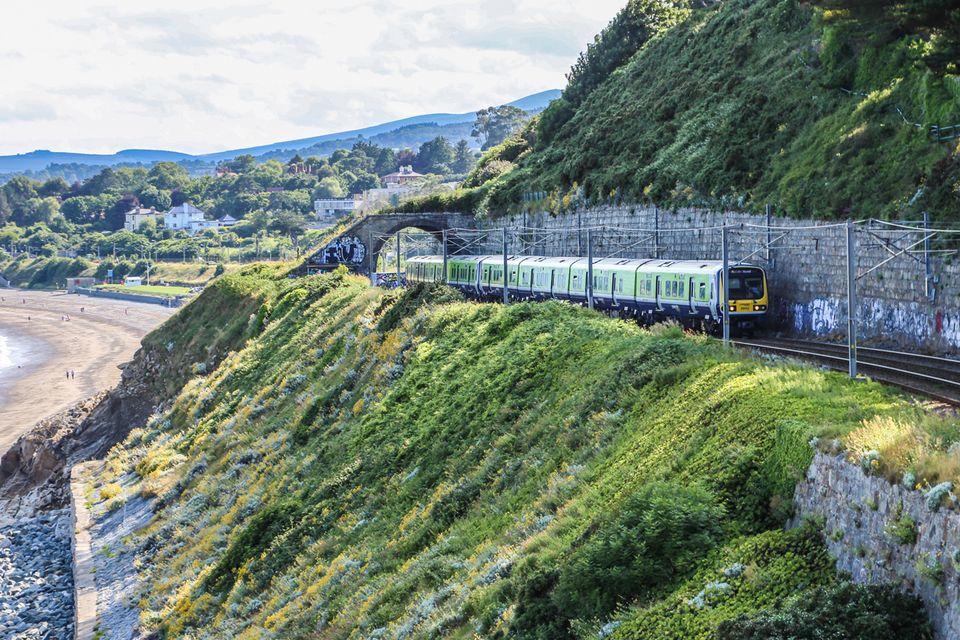
There were definitely some hiccups along the way. Getting the state management right took a bit of trial and error. I also had some issues with the layout initially, but playing around with Expanded
and Flexible
widgets eventually got it looking how I wanted. And, of course, debugging those pesky Flutter errors is always an adventure.
In the end, "dart town" isn't anything fancy. It's just a simple app that lets you add and remove building names. But it was a really helpful exercise in understanding the basics of Flutter, state management, and UI layout. Plus, it was kinda fun to see my little town grow with each building I added!
Lessons learned:
- Stateful widgets are your friend when you need to manage data and update the UI.
- is great for displaying lists of things.
Dismissible
is a surprisingly easy way to add swipe-to-delete functionality.- Debugging Flutter is a skill in itself.
I'm thinking of adding some more features, like letting you change the color of the buildings, or maybe even add little icons. But for now, I'm happy with my little "dart town."